Web graphics
a large creative palette
Created with CSS 3D Slideshow - by Hakim El Hattab
About this presentation
This presentation was given at the W3Conf 2011 conference in Seattle. The slides are supporting the presentation, and do not have enough textual information to be understood by themselves.
You can see a video of the presentation here (look for the "Web Graphics - A large creative palette" video thumbnail).
The presentation is written in HTML5 and uses some features that are only supported by the most modern browsers. It was developed with the latest Chrome browser version.
You can navigate with the left and right arrow keys and with the up/down keys to drill into sections.
Simple Graphics - SVG
Simple Graphics - SVG (code)
<svg width="600" height="400" viewBox="0 0 600 400"> <circle cx="300" cy="200" r="175" stroke="#2263B2" stroke-width="50" fill="#1780FF" /> <circle r="75" transform="translate(300,200)" stroke="#B1CC27" stroke-width="50" fill="#96B207" /> </svg>
Simple Graphic - Canvas
Simple Graphics - Canvas
<canvas id="c1" width="600px" height="400px"></canvas> <script> (function () { var canvas = document.getElementById('c1'); var ctx = canvas.getContext('2d'); ctx.fillStyle = '#96B207'; ctx.strokeStyle = '#B1CC27'; ctx.lineWidth = 50; ctx.beginPath(); ctx.arc(300, 200, 175, 0, 360, false); ctx.fill(); ctx.stroke(); ctx.fillStyle = '#1780FF'; ctx.strokeStyle = '#2263B2'; ctx.beginPath(); ctx.translate(300, 200); ctx.arc(0, 0, 75, 0, 360, false); ctx.fill(); ctx.stroke(); })(); </script>
Graphics Rendering
What is rendered?
- Graphical objects: images, text, basic and complex shapes
- Elements in SVG
- Drawing calls in Canvas
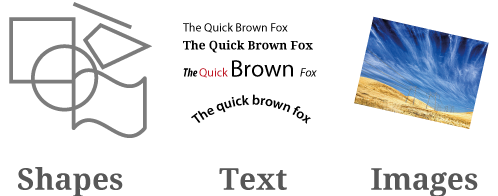
How are objects rendered?
- Controlled by the rendering context
- Canvas: held in the canvas context object
- SVG: set by the computed values on graphical objects and structural objects
What is in the rendering context?
- transform
- fill style: solid color, gradient, pattern
- stroking style: stroke paint and stroke properties
- text and font properties
What is in the rendering context?
- opacity
- filter and composite
- masks and clips
- markers
The sophistication of the rendering context varies between SVG and canvas
Interactivity
Animation
Styling
Eventing
- In canvas, we are responsible for mapping an event to its graphical target. Frameworks are handy.
- In SVG, events work as in HTML with the pointer-events property
pointer-events
pointer-events
<circle id="cn" r="30" stroke-width="15" stroke="[?]" fill="[?]" visibility="[?]" pointer-events="[?]"/> <text y="60">[label] <animateTransform attributeName="transform" type="scale" values="1 1;0 1;1 1" begin="cn.click" dur="0.5s"> </text>
Scripting
- canvas: some additional APIs for focus management, caret management and text metrics
- svg: DOM APIs for all features, including interaction with the text engine (advanced text metrics), the animation engine and events
SVG Path API
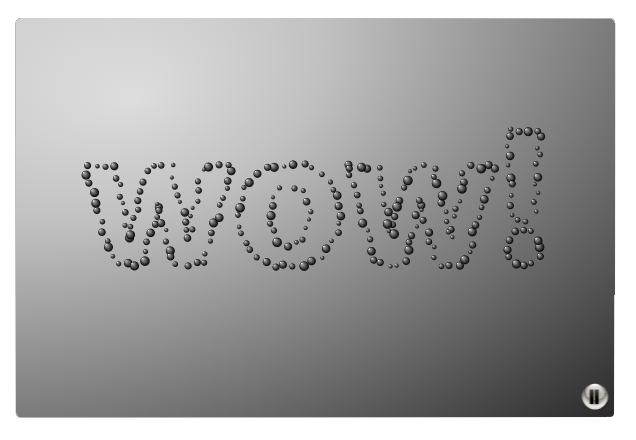
Animation
- Scripted animation are always an option
- SVG offers SMIL animation, and implementations are starting to support CSS animations and transitions on SVG properties
Declarative Animation
click on the cross to see it morph into a check mark
Declarative Animation
<path id="morph" fill="url(#blue-grad)"
transform="translate(0,20)"
filter='url(#drop-shadow)'
d="M29,1.5....,29,1.5z">
<animate attributeName="d"
to="M12,30...12,30z"
begin="cross.click"
dur="1s"
fill="freeze"/>
</path>
Timing and Synchronization
click on left-most sphere to start the animation
Timing and Synchronization
<g id="golden-sphere">
<line id="wire" ... />
<circle id="attach" />
<circle id="sphere" />
<animateTransform
attributeName="transform"
type="rotate" values="0;-90;0"
begin="graySphereAnim.end"
dur="1s"
keyTimes="0;0.5;1"
keySplines="0 1 1 1;1 0 1 1"
calcMode="spline" />
</g>
Styling
SVG can be styled with CSS
like any HTML content.
click on the image to see the demo
Multi-media
Integration
Audio
- Use the html
<audio>
tag (orAudio
JavaScript objects) - New work on the Web audio API
Audio & Graphics
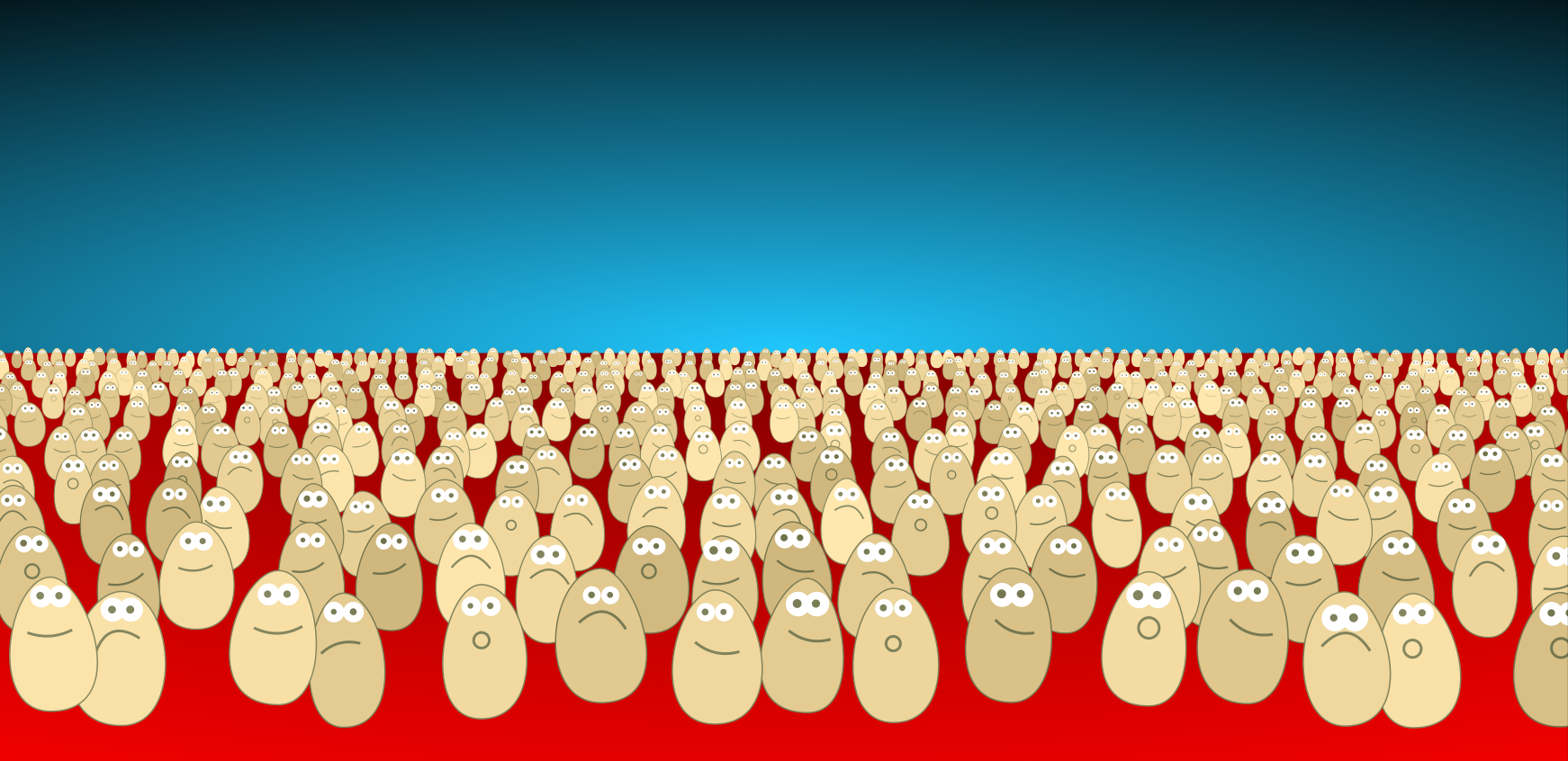
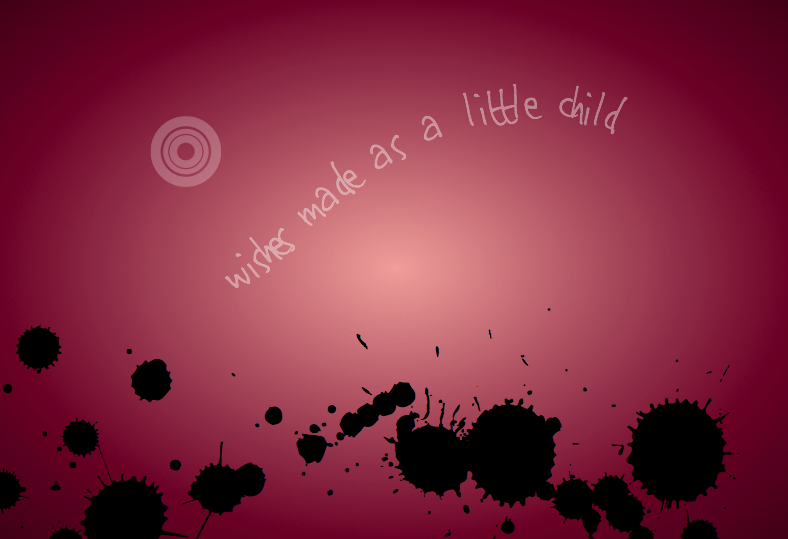
click on the images to see the demos
Video and Canvas
var ctx = canvas.getContext('2d'); var video = getElementById('myVideo'); ctx.drawImage(video, 0, 0, video.videoWidth, video.videoHeight);
Video in SVG
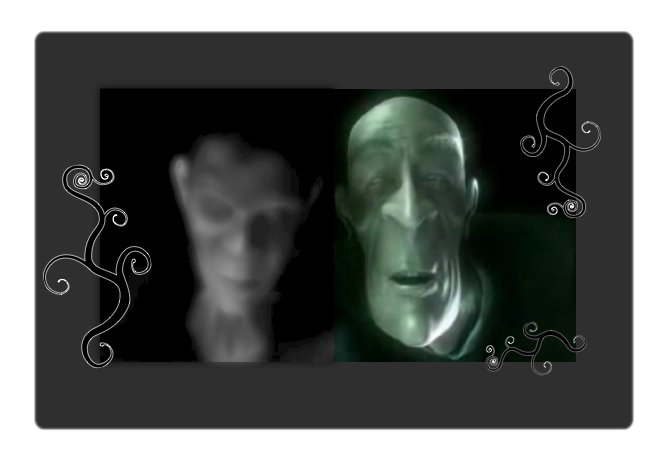
click on the image to see the demo (Firefox required)
HTML5 Graphics Bridges
HTML5 in SVG
HTML5 in SVG
<svg width="600" height="400">
<defs>...</defs>
<g id="cloud"
filter="url(#drop-shadow)">
<path d="M515.94,131.974 ..."/>
<foreignObject
x="144" y="78"
width="304" height="183">
<body xmlns=".../xhtml">
<h2>thought</h2>
<div>...</div>
</body>
</foreignObject>
</g>
</svg>
Canvas in SVG - Take 1
click on the 'draw in canvas' text
Canvas in SVG - Take 2
click on the image to see the demo
About
canvas.toBlob(cb);
- Specified in the HTMLCanvasElement specification
- Not widely implemented yet
- Will be much more efficient than Base64 encoded data to exchange pixel data.
SVG in Canvas
click on black rectangle to start / stop the animation
icons from the the noun project
What next?
- More content & web sites
- Rendering arbitrary HTML content in Canvas and
element()
function - Filter effects and CSS shaders for pixel manipulation
- Videos and filter effects is especially promissing
- Advanced typography
Typography on the Web: attend Christopher Slye's presentation
Attributions
- Slide tool by Hakim El Hattab with minor modifications.
- Palette demo done with D3.js by Michael Bostock
- Icons from the noun project
- Video from the Elephants dream movie.
- Music by Anika Williams
- Fonts: